Homework 2: Several Little Mysteries
Due: Tuesday September 17 2019, 9:00PM.
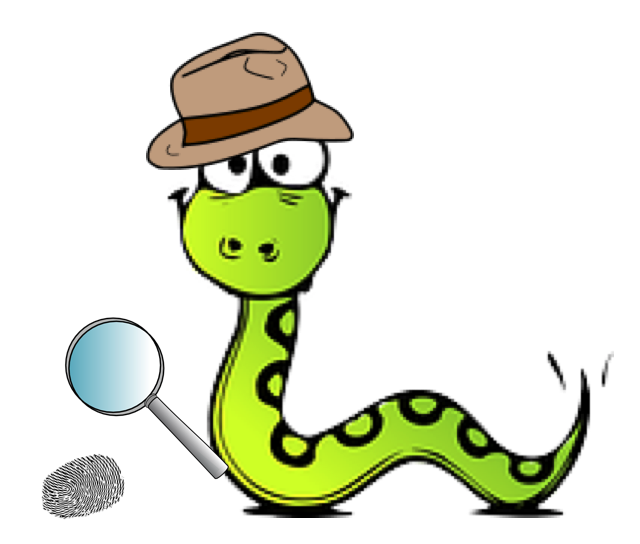
Handin
- Make sure to read through the entire handout before starting. This is crucial for knowing what to expect from the assignment, while estimating how much time it may take. For example, in this assignment there is a reading portion listed under the README section. However, because that section is at the very end, if you wait until the last minute, you may miss it! I encourage you to read everything and plan ahead!
- Download your file(s) and make sure the follow the naming convention listed in the assignment handout. Hand in your work on Gradescope. Look at this guide for directions.
- You may submit as many times as you want. Only your latest submission will be graded. This means that if you submit after the deadline, you will be using a late day – so do NOT submit after the deadline unless you plan on using late days.
- Do not put your name anywhere in any of the handin files. Doing so will result in a deduction.
- The README template can be found here
After completing the homework, you will submit:
README.txt
more-images.arr
doors-and-locks.arr
mystery-scene.arr
If you are using late days, make sure to make a note of that in your readme. Remember, you may only use a maximum of 3 late days per assignment. If the assignment is late (and you do NOT have anymore late days) no credit will be given.
Setup
You will need a text editor to edit your README. Link to template provided above. Any text editor is fine, and your laptop should already have one (TextEdit on Mac, NotePad on Windows). You could also install a more powerful text editor (such as Atom, instructions here), but this is not required. If you are still having a hard time, come to hours or post on Campuswire!
Helpful Things
Documentation
- Pyret Documentation, accessible from the Pirate button in the top left corner of code.pyret.org. The Image and String documentation will likely be the most useful for this assignment.
- Pyret Testing Design and Clarity Guide, while the inital portion (testing) may not be necessary for this assignment, I highly encourage you to read the latter. This will be important for some parts of this assignment. If there is anything that you find unclear, come to hours or post on Campuswire (we are happy to help)!
Useful Functions
-
not
is a builtin function that takes a Boolean, and inverts it (not(true)
→ false
and not(false)
→ true
)
-
==
is an equality test; 3 == 3
→ true
and 3 == 4
→ false
.
-
<>
is an inequality test; 3 <> 4
→ true
and 3 <> 3
→ false
The Assignment
More Images
Someone left some clues at the detective station but Detective Pikachu and Velma lost the clues. From memory, they remember it looking like these two pictures:
Picture One

Picture Two

They have asked you to recreate it so they can show it to the other folks at the station. Fill in the functions such that the functions:
picture-one
will return the a blue rain drop and a red bow next to each other
picture-two
will return a yellow star with a red outline and an orange goldfish
This homework of the problem will follow a similar structure to last weeks. That is, we will not penalize you for using different colors as long as it follows the same pattern as the images. So, if you decide you want to fish to be purple instead of orange, that is fine, but you may not change the colors of the fish. For example, you will lose points if you decided to make the body of the fish purple and the tail orange.
Major hint:
We have left an example in the stencil code to help you think about how to structure your code and recreate the picture. To build the lollipop example, try breaking the whole image into smaller chunks.
Suppose we had to create the lollipop. We see that there is some circular portion, which is supposed to represent the candy part, and there is a rectangle shape, which is supposed to represent the handle. So, we can break this problem into two parts. The candy part and the handle part. Once creating each lollipop piece, we can put it together. I recommend using the overlay function, which will allow you to place shapes on top of each other.

(Optional) Those images from above (picture one and two) are supposed to represent pictograms. Can you guess what they are supposed to represent?
A Series of Doors and Locks
Velma and Detective Pikachu need to get to their next destination. In order to get to their next destination, they must fix a series of doors that are blocking their way. Currently the doors never open. However, each door should only open in certain instances.
The doors are represented as functions. These functions will take in Boolean inputs, and depending on those inputs, will either open or close. When a function returns true, the door is opened. When a function returns false, a door is not opened. Help Velma and Detective Pikachu fix the doors!
Here are two examples:
# This door should always open, regardless of the input.
# Do you see why?
fun door-a(input :: Boolean) -> Boolean:
true
end
# This door will only open if the input is true, if the input
# is not true, then it will return false and will not open.
fun door-b(input :: Boolean) -> Boolean:
input
end
Open doors-and-locks.arr using the link above, and get each door to work.
Here is a quick summary of what each door should and shouldn’t do:
door-one
should return the opposite of the input. That is, if the input is true then return false, and if it’s false then return true.
door-two
should return true if input-a is not the same as input-b
door-three
should return true if input-a is equal to the opposite of input-b and if the result of that is not equal to input-c
door-four
should return true only when one of the inputs is true, but you may not use the <> sign
Advice: We’ve provided some test cases, but they are not exhaustive. We encourage you to add more so you gain a better understanding of output you are expecting. Adding additional test cases is optional, you will not be graded on what test cases you add for this problem.
Mystery Scene
As a gesture of gratitude for fixing the doors and mystery functions and cleaning up her code, Jennifer shares a picture of a mystery scene with you. She tells you that there were three detectives and two clues at the scene. However, the scene is represented as code.
Look at the file linked above and run the functiondraw-game-screen
with a few different numerical inputs to see how it works.
include image
finger-print-url = 'https://static01.nyt.com/images/2012/06/10/magazine/10wmt/mag-10WMT-t_CA0-jumbo.jpg'
magnifying-glass-url = 'https://image.flaticon.com/icons/png/512/63/63322.png'
s1 = image-url(finger-print-url)
s2 = image-url(magnifying-glass-url)
fun draw-game-screen(position):
underlay-xy(
underlay-xy(circle(180, 'solid', 'blue'), position, 500, s1),
30, 20,
above(
beside(
flip-vertical(s2),
beside(
rectangle(100, 1, 'solid', 'transparent'),
flip-vertical(s2))),
above(
rectangle(1, 50, 'solid', 'transparent'),
flip-vertical(s2))))
end
However, this picture script does not follow your CS111 style guide!
-
Add a type annotation to the function.
-
Add a docstring to the function. Good documentation includes:
-
A description of the input(s).
-
A description of the output.
-
Any restrictions on the input(s).
-
Clean up this function; keep the same function name, draw-game-screen
.
Note: the output image does not have to be exactly the same, but it should be similar.
Tip: Sometimes it can be very hard to read code. It can help to try changing some numbers around and seeing what effect that has.
-
Numbers used for positioning should not be hard-coded, but should be given a useful name. For example, CIRCLE-RADIUS + PADDING
is clearer than 30 + 5
.
-
Numbers that are based on their context should be computed based on their context. The image-width
and image-height
functions may be helpful for this.
-
Remove repeated computation using either helper functions or constants.
-
Rename constants if they are unclearly named.
-
Add short comments for lines that may not be intuitive.
-
When you are making constants, think about whether to put them inside or outside of the function. In general, you should only have constants inside of the function if they depend on the inputs.
pi = 3.14
fun area(R):
pi * num-sqr(R)
end
is cleaner than
fun area(R):
pi = 3.14
pi * num-sqr(R)
end
There is no need to redefine pi
every single time area
is used; pi
never changes.
README.txt
Fill out your README as usual, but this week there will be an additional portion, which is the ethics assignment.
Ethics Assignment
Additional responses required for this week will pertain to the ethics assignment.
Data Perspectives: Algorithmic Bias
Beyond teaching you some technical skills around computing and data, CS111 also wants to help you think about some of the broader societal issues surrounding data and computing. To this end, we will have a few short reading assignments that ask you to reflect on implications and possible principles of working with computing and data. This is the first such assignment.
We estimate this assignment will take roughly an hour.
Topic: Algorithmic Bias
You’ll put your answers in the README, clearly marking which answers belong to which questions. Your answer to each question should be a few sentences long. Your answers should be clear, concise, and demonstrate serious reflection, but there are no right or wrong answers. There will be an opportunity to discuss this topic in class.
Racial bias in algorithmic decision-making is (deservedly) getting a lot of attention. Read this Propublica article on racial bias in algorithms used for prison sentencing and recidivism. Also read this MIT Technology Review article about what causes algorithmic bias and why it’s so hard to fix.
Answer the following questions:
- Use the framework of the MIT Technology Review article to understand the issues described in the Propublica article: Which of the three causes of algorithmic bias do you believe plays the largest role in the issues described in the Propublic article? Which of the four challenges do you believe poses the most difficulty for mitigating the algorithmic bias issues described in the Propublic article?
- With all of the problems these predictive technologies have yielded, what’s the appeal to using them?
- Given the difficulty of fixing algorithmic bias, are there any contexts where you believe predictive algorithms should never be used? Explain why.
- Think of a project or potential project in which you might use computation over data to help answer questions. This could be an issue you’ve discussed in another course, something you’ve read about in the media, an issue you are engaged with through extracurricular efforts, or so on. In a few sentences, describe the project, talk about how bias might emerge, how you might prevent it, and what you might do to gain trust in the decisions rendered by the program. Our goal is to have you try to contextualize these readings in a problem that is meaningful to you.
Grading
We will check that your responses:
- are related to the readings,
- demonstrate evidence of critical thinking and reflection
- are well written, rather than being full of spelling and grammar mistakes.
Brown University CSCI 0111 (Fall 2019)
Do you have feedback? Fill out this form.
Homework 2: Several Little Mysteries
Due: Tuesday September 17 2019, 9:00PM.
Handin
After completing the homework, you will submit:
README.txt
more-images.arr
doors-and-locks.arr
mystery-scene.arr
If you are using late days, make sure to make a note of that in your readme. Remember, you may only use a maximum of 3 late days per assignment. If the assignment is late (and you do NOT have anymore late days) no credit will be given.
Setup
You will need a text editor to edit your README. Link to template provided above. Any text editor is fine, and your laptop should already have one (TextEdit on Mac, NotePad on Windows). You could also install a more powerful text editor (such as Atom, instructions here), but this is not required. If you are still having a hard time, come to hours or post on Campuswire!
Helpful Things
Documentation
Useful Functions
not
is a builtin function that takes a Boolean, and inverts it (not(true)
→false
andnot(false)
→true
)==
is an equality test;3 == 3
→true
and3 == 4
→false
.<>
is an inequality test;3 <> 4
→true
and3 <> 3
→false
The Assignment
More Images
Someone left some clues at the detective station but Detective Pikachu and Velma lost the clues. From memory, they remember it looking like these two pictures:
Picture One

Picture Two

They have asked you to recreate it so they can show it to the other folks at the station. Fill in the functions such that the functions:
picture-one
will return the a blue rain drop and a red bow next to each otherpicture-two
will return a yellow star with a red outline and an orange goldfishThis homework of the problem will follow a similar structure to last weeks. That is, we will not penalize you for using different colors as long as it follows the same pattern as the images. So, if you decide you want to fish to be purple instead of orange, that is fine, but you may not change the colors of the fish. For example, you will lose points if you decided to make the body of the fish purple and the tail orange.
Major hint:
We have left an example in the stencil code to help you think about how to structure your code and recreate the picture. To build the lollipop example, try breaking the whole image into smaller chunks.
Suppose we had to create the lollipop. We see that there is some circular portion, which is supposed to represent the candy part, and there is a rectangle shape, which is supposed to represent the handle. So, we can break this problem into two parts. The candy part and the handle part. Once creating each lollipop piece, we can put it together. I recommend using the overlay function, which will allow you to place shapes on top of each other.
(Optional) Those images from above (picture one and two) are supposed to represent pictograms. Can you guess what they are supposed to represent?
A Series of Doors and Locks
Velma and Detective Pikachu need to get to their next destination. In order to get to their next destination, they must fix a series of doors that are blocking their way. Currently the doors never open. However, each door should only open in certain instances.
The doors are represented as functions. These functions will take in Boolean inputs, and depending on those inputs, will either open or close. When a function returns true, the door is opened. When a function returns false, a door is not opened. Help Velma and Detective Pikachu fix the doors!
Here are two examples:
Open doors-and-locks.arr using the link above, and get each door to work.
Here is a quick summary of what each door should and shouldn’t do:
door-one
should return the opposite of the input. That is, if the input is true then return false, and if it’s false then return true.door-two
should return true if input-a is not the same as input-bdoor-three
should return true if input-a is equal to the opposite of input-b and if the result of that is not equal to input-cdoor-four
should return true only when one of the inputs is true, but you may not use the <> signAdvice: We’ve provided some test cases, but they are not exhaustive. We encourage you to add more so you gain a better understanding of output you are expecting. Adding additional test cases is optional, you will not be graded on what test cases you add for this problem.
Mystery Scene
As a gesture of gratitude for fixing the doors and mystery functions and cleaning up her code, Jennifer shares a picture of a mystery scene with you. She tells you that there were three detectives and two clues at the scene. However, the scene is represented as code.
Look at the file linked above and run the function
draw-game-screen
with a few different numerical inputs to see how it works.However, this picture script does not follow your CS111 style guide!
Add a type annotation to the function.
Add a docstring to the function. Good documentation includes:
A description of the input(s).
A description of the output.
Any restrictions on the input(s).
Clean up this function; keep the same function name,
draw-game-screen
.Note: the output image does not have to be exactly the same, but it should be similar.
Tip: Sometimes it can be very hard to read code. It can help to try changing some numbers around and seeing what effect that has.
Numbers used for positioning should not be hard-coded, but should be given a useful name. For example,
CIRCLE-RADIUS + PADDING
is clearer than30 + 5
.Numbers that are based on their context should be computed based on their context. The
image-width
andimage-height
functions may be helpful for this.Remove repeated computation using either helper functions or constants.
Rename constants if they are unclearly named.
Add short comments for lines that may not be intuitive.
When you are making constants, think about whether to put them inside or outside of the function. In general, you should only have constants inside of the function if they depend on the inputs.
is cleaner than
There is no need to redefine
pi
every single timearea
is used;pi
never changes.README.txt
Fill out your README as usual, but this week there will be an additional portion, which is the ethics assignment.
Ethics Assignment
Additional responses required for this week will pertain to the ethics assignment.
Data Perspectives: Algorithmic Bias
Beyond teaching you some technical skills around computing and data, CS111 also wants to help you think about some of the broader societal issues surrounding data and computing. To this end, we will have a few short reading assignments that ask you to reflect on implications and possible principles of working with computing and data. This is the first such assignment.
We estimate this assignment will take roughly an hour.
Topic: Algorithmic Bias
You’ll put your answers in the README, clearly marking which answers belong to which questions. Your answer to each question should be a few sentences long. Your answers should be clear, concise, and demonstrate serious reflection, but there are no right or wrong answers. There will be an opportunity to discuss this topic in class.
Racial bias in algorithmic decision-making is (deservedly) getting a lot of attention. Read this Propublica article on racial bias in algorithms used for prison sentencing and recidivism. Also read this MIT Technology Review article about what causes algorithmic bias and why it’s so hard to fix.
Answer the following questions:
Grading
We will check that your responses: